How to Import CSV file into Mysql using PHP
1 min read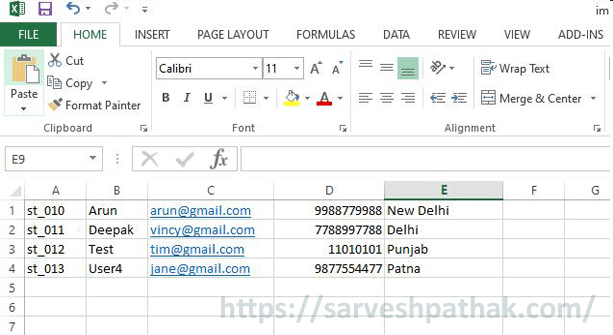
How to Import CSV file into Mysql using PHP
Here we will understand the following steps to How to Import CSV file into Mysql using PHP
- Create MySql Table.
- Create PHP Form for CSV File Upload.
- Insert all the records from CSV in the Database.
- Show Inserted Records.
Step 1- Create MySql Table using Following Command.
CREATE TABLE `student` (
`id` int(11) NOT NULL,
`name` varchar(100) NOT NULL,
`email` varchar(100) NOT NULL,
`phone` varchar(100) NOT NULL,
`city` varchar(100) NOT NULL,
`student_id` varchar(20) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
How to Import CSV file into Mysql using PHP
Step 2- Create a form for CSV File Upload
<form class="form-horizontal" action="" method="post"
name="frmCSVImport" id="frmCSVImport"
enctype="multipart/form-data">
<div class="input-row">
<label class="col-md-4 control-label">Choose CSV
File</label> <input type="file" name="file"
id="file" accept=".csv">
<button type="submit" id="submit" name="import"
class="btn-submit">Import</button>
<br />
</div>
</form>
How to Import CSV file into Mysql using PHP
Step 3- Insert all the records from CSV in the Database after form Submit.
<?php
$servername = "localhost";
$email = "root";
$phone = "";
$dbname = "crud";
$con = mysqli_connect($servername, $email, $phone, $dbname);
if (!$con) {
die("Connection failed: " . mysqli_connect_error());
}
if (isset($_POST["import"])) {
$fileName = $_FILES["file"]["tmp_name"];
if ($_FILES["file"]["size"] > 0) {
$file = fopen($fileName, "r");
while (($column = fgetcsv($file, 10000, ",")) !== FALSE) {
$student_id = "";
if (isset($column[0])) {
$student_id = mysqli_real_escape_string($con, $column[0]);
}
$name = "";
if (isset($column[1])) {
$name = mysqli_real_escape_string($con, $column[1]);
}
$email = "";
if (isset($column[2])) {
$email = mysqli_real_escape_string($con, $column[2]);
}
$phone = "";
if (isset($column[3])) {
$phone = mysqli_real_escape_string($con, $column[3]);
}
$city = "";
if (isset($column[4])) {
$city = mysqli_real_escape_string($con, $column[4]);
}
$sqlInsert = "INSERT into student (name,email,phone,city,student_id)values ('$name','$email', '$phone','$city','$student_id')";
$run=mysqli_query($con,$sqlInsert);
if($run)
{
$type = "success";
$message = "CSV Data Imported into the Database";
} else {
$type = "error";
$message = "Problem in Importing CSV Data";
$message=$sqlInsert;
}
}
}
}
?>
How to Import CSV file into Mysql using PHP
Step 4 – Show All Records
<table class="record" >
<thead>
<tr>
<th>Student ID</th>
<th>Student Name</th>
<th>Student Email</th>
<th>Contact No</th>
<th>City</th>
</tr>
</thead>
<?php $sqlSelect = "SELECT * FROM student";
$query=mysqli_query($con,$sqlSelect);
$cnt=1;
while($row=mysqli_fetch_array($query))
{
?>
<tbody>
<tr>
<td><?php echo $row['student_id']; ?></td>
<td><?php echo $row['name']; ?></td>
<td><?php echo $row['email']; ?></td>
<td><?php echo $row['phone']; ?></td>
<td><?php echo $row['city']; ?></td>
</tr>
<?php
}
?>
</tbody>
</table>
How to Import CSV file into Mysql using PHP
Click > Download Complete Source Code
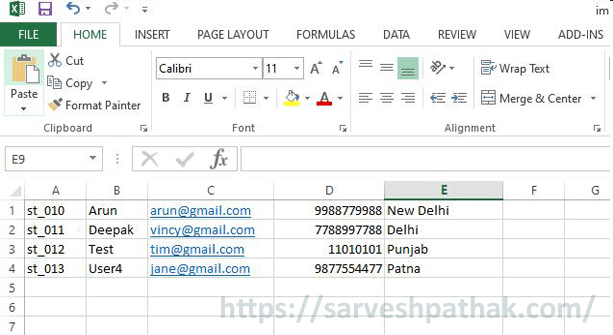
This paragraph is really a nice one it helps new net users, who are wishing for blogging. Anabelle Arney Iy