Integrate Sweetalert 2 in PHP & MySQL Using Ajax Download Free Example
1 min read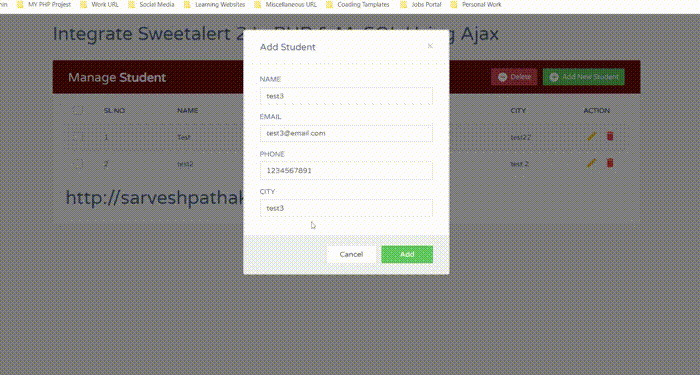
Integrate Sweetalert 2 In PHP & MySQL Using Ajax
In this tutorial, We will learn How to Integrate Sweetalert 2 in PHP & MySQL using Ajax. Sweet alert 2 allows developers to customize the alert box as per your web applications. The look and feel of the Sweetalert 2 are amazing and many developers love it. So in this article, I will show how to integrate it easily into your crud application in PHP and MySQL using Bootstrap Modal Popup and AJAX.
Create a Database and a table with the following structure to Create Crud in PHP and MySQL using Bootstrap Modal Popup and AJAX
CREATE TABLE `student` (
`id` int(11) NOT NULL,
`name` varchar(100) NOT NULL,
`email` varchar(100) NOT NULL,
`phone` varchar(100) NOT NULL,
`city` varchar(100) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
ADD PRIMARY KEY (`id`);
ALTER TABLE `student`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=21;
COMMIT;
Connect to the Database: bd.php
<?php
$servername = "localhost";
$username = "root";
$password = "Dopm";
$dbname = "tutorial";
$conn = mysqli_connect($servername, $username, $password, $dbname);
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
?>Integrate Sweetalert 2 in PHP
Create Index.php to Integrate Sweetalert
This page will perform insert, update and delete with Sweetalert.
<?php
include 'bd.php';
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>User Data</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto|Varela+Round">
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<link rel="stylesheet" href="style.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<script src="https://unpkg.com/sweetalert/dist/sweetalert.min.js"></script>
<script src="index.js"></script>
</head>
<body>
<div class="container">
<h1> How to Integrate Sweetalert 2 in PHP & MySQL using Ajax </h1>
<p id="success"></p>
<div class="table-wrapper">
<div class="table-title">
<div class="row">
<div class="col-sm-6">
<h2>Manage <b>Student</b></h2>
</div>
<div class="col-sm-6">
<a href="#addEmployeeModal" class="btn btn-success" data-toggle="modal"><i class="material-icons"></i> <span>Add New Student</span></a>
<a href="JavaScript:void(0);" class="btn btn-danger" id="delete_multiple"><i class="material-icons"></i> <span>Delete</span></a>
</div>
</div>
</div>
<table class="table table-striped table-hover">
<thead>
<tr>
<th>
<span class="custom-checkbox">
<input type="checkbox" id="selectAll">
<label for="selectAll"></label>
</span>
</th>
<th>SL NO</th>
<th>NAME</th>
<th>EMAIL</th>
<th>PHONE</th>
<th>CITY</th>
<th>ACTION</th>
</tr>
</thead>
<tbody>
<?php
$result = mysqli_query($conn,"SELECT * FROM student");
$i=1;
while($row = mysqli_fetch_array($result)) {
?>
<tr id="<?php echo $row["id"]; ?>">
<td>
<span class="custom-checkbox">
<input type="checkbox" class="user_checkbox" data-user-id="<?php echo $row["id"]; ?>">
<label for="checkbox2"></label>
</span>
</td>
<td><?php echo $i; ?></td>
<td><?php echo $row["name"]; ?></td>
<td><?php echo $row["email"]; ?></td>
<td><?php echo $row["phone"]; ?></td>
<td><?php echo $row["city"]; ?></td>
<td>
<a href="#editEmployeeModal" class="edit" data-toggle="modal">
<i class="material-icons update" data-toggle="tooltip"
data-id="<?php echo $row["id"]; ?>"
data-name="<?php echo $row["name"]; ?>"
data-email="<?php echo $row["email"]; ?>"
data-phone="<?php echo $row["phone"]; ?>"
data-city="<?php echo $row["city"]; ?>"
title="Edit"></i>
</a>
<a href="#deleteEmployeeModal" class="delete" data-id="<?php echo $row["id"]; ?>" data-toggle="modal"><i class="material-icons" data-toggle="tooltip"
title="Delete"></i></a>
</td>
</tr>
<?php
$i++;
}
?>
</tbody>
</table>
<h1> http://sarveshpathak.com/</h1>
</div>
</div>
<!-- Add Modal HTML -->
<div id="addEmployeeModal" class="modal fade">
<div class="modal-dialog">
<div class="modal-content">
<form id="user_form">
<div class="modal-header">
<h4 class="modal-title">Add Student</h4>
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
</div>
<div class="modal-body">
<div class="form-group">
<label>NAME</label>
<input type="text" id="name" name="name" class="form-control" required>
</div>
<div class="form-group">
<label>EMAIL</label>
<input type="email" id="email" name="email" class="form-control" required>
</div>
<div class="form-group">
<label>PHONE</label>
<input type="phone" id="phone" name="phone" class="form-control" required>
</div>
<div class="form-group">
<label>CITY</label>
<input type="city" id="city" name="city" class="form-control" required>
</div>
</div>
<div class="modal-footer">
<input type="hidden" value="1" name="type">
<input type="button" class="btn btn-default" data-dismiss="modal" value="Cancel">
<button type="button" class="btn btn-success" id="btn-add">Add</button>
</div>
</form>
</div>
</div>
</div>
<!-- Edit Modal HTML -->
<div id="editEmployeeModal" class="modal fade">
<div class="modal-dialog">
<div class="modal-content">
<form id="update_form">
<div class="modal-header">
<h4 class="modal-title">Edit Student</h4>
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
</div>
<div class="modal-body">
<input type="hidden" id="id_u" name="id" class="form-control" required>
<div class="form-group">
<label>Name</label>
<input type="text" id="name_u" name="name" class="form-control" required>
</div>
<div class="form-group">
<label>Email</label>
<input type="email" id="email_u" name="email" class="form-control" required>
</div>
<div class="form-group">
<label>PHONE</label>
<input type="phone" id="phone_u" name="phone" class="form-control" required>
</div>
<div class="form-group">
<label>City</label>
<input type="city" id="city_u" name="city" class="form-control" required>
</div>
</div>
<div class="modal-footer">
<input type="hidden" value="2" name="type">
<input type="button" class="btn btn-default" data-dismiss="modal" value="Cancel">
<button type="button" class="btn btn-info" id="update">Update</button>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
index.js Integrate Sweetalert 2 in PHP & MySQL Using Ajax
//Integrate Sweetalert 2 in PHP & MySQL using Ajax on Add New Record
$(document).on("click", "#btn-add", function (e) {
var data = $("#user_form").serialize();
$.ajax({
data: data,
type: "post",
url: "save.php",
success: function (dataResult) {
var dataResult = JSON.parse(dataResult);
if (dataResult.statusCode == 200) {
$("#addEmployeeModal").modal("hide");
swal("Good job!", "Data Saved !", "success");
setTimeout(200000);
location.reload();
} else if (dataResult.statusCode == 201) {
//alert(dataResult);
swal("Error!", "Could'not be saved !", "warnings");
setTimeout(2000);
}
},
});
});
//Update in Sweet alert PHP MySQL
$(document).on("click", ".update", function (e) {
var id = $(this).attr("data-id");
var name = $(this).attr("data-name");
var email = $(this).attr("data-email");
var phone = $(this).attr("data-phone");
var city = $(this).attr("data-city");
$("#id_u").val(id);
$("#name_u").val(name);
$("#email_u").val(email);
$("#phone_u").val(phone);
$("#city_u").val(city);
});
//Sweet alert PHP MySQL
$(document).on("click", "#update", function (e) {
var data = $("#update_form").serialize();
$.ajax({
data: data,
type: "post",
url: "save.php",
success: function (dataResult) {
var dataResult = JSON.parse(dataResult);
if (dataResult.statusCode == 200) {
$("#editEmployeeModal").modal("hide");
swal("Good job!", "Data Updated Successfully!", "success");
setTimeout(2000);
location.reload();
} else if (dataResult.statusCode == 201) {
swal("Error!", "Could'not be Updated !", "Warnings");
setTimeout(2000);
}
},
});
});
//Delete using sweet alert in PHP MySQL
$(document).on("click", ".delete", function () {
var recordid = $(this).attr("data-id");
swal({
title: "Are you sure?",
text: "Once deleted, you will not be able to recover this Record !",
icon: "warning",
buttons: true,
dangerMode: true,
}).then((willDelete) => {
if (willDelete) {
$.ajax({
url: "save.php",
type: "POST",
cache: false,
data: {
type: 3,
id: recordid,
},
success: function (dataResult) {
location.reload();
$("#" + dataResult).remove();
},
});
swal("Record Deleted successfully !", {
icon: "success",
});
} else {
swal("Your record is safe");
}
});
});
//Multiple Delete using sweet alert in PHP MySQL
$(document).on("click", "#delete_multiple", function () {
var user = [];
$(".user_checkbox:checked").each(function () {
user.push($(this).data("user-id"));
});
if (user.length <= 0) {
alert("Please select records.");
} else {
swal({
title: "Are you sure?",
text: "Once deleted, you will not be able to recover this Record !",
icon: "warning",
buttons: true,
dangerMode: true,
}).then((willDelete) => {
if (willDelete) {
var selected_values = user.join(",");
//console.log(selected_values);
$.ajax({
type: "POST",
url: "save.php",
cache: false,
data: {
type: 4,
id: selected_values,
},
success: function (response) {
var ids = response.split(",");
for (var i = 0; i < ids.length; i++) {
$("#" + ids[i]).remove();
}
},
});
location.reload();
swal("Record Deleted successfully !", {
icon: "success",
});
} else {
swal("Your imaginary file is safe!");
}
});
}
});
$(document).ready(function () {
$('[data-toggle="tooltip"]').tooltip();
var checkbox = $('table tbody input[type="checkbox"]');
$("#selectAll").click(function () {
if (this.checked) {
checkbox.each(function () {
this.checked = true;
});
} else {
checkbox.each(function () {
this.checked = false;
});
}
});
checkbox.click(function () {
if (!this.checked) {
$("#selectAll").prop("checked", false);
}
});
});
save.php Integrate Sweetalert 2 in PHP & MySQL using Ajax
<?php
include 'bd.php';
if(count($_POST)>0){
if($_POST['type']==1){
$name=$_POST['name'];
$email=$_POST['email'];
$phone=$_POST['phone'];
$city=$_POST['city'];
$sql = "INSERT INTO `student`( `name`, `email`,`phone`,`city`)
VALUES ('$name','$email','$phone','$city')";
if (mysqli_query($conn, $sql)) {
echo json_encode(array("statusCode"=>200));
}
else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
mysqli_close($conn);
}
}
if(count($_POST)>0){
if($_POST['type']==2){
$id=$_POST['id'];
$name=$_POST['name'];
$email=$_POST['email'];
$phone=$_POST['phone'];
$city=$_POST['city'];
$sql = "UPDATE `student` SET `name`='$name',`email`='$email',`phone`='$phone',`city`='$city' WHERE id=$id";
if (mysqli_query($conn, $sql)) {
echo json_encode(array("statusCode"=>200));
}
else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
mysqli_close($conn);
}
}
if(count($_POST)>0){
if($_POST['type']==3){
$id=$_POST['id'];
$sql = "DELETE FROM `student` WHERE id=$id ";
if (mysqli_query($conn, $sql)) {
echo $id;
}
else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
mysqli_close($conn);
}
}
if(count($_POST)>0){
if($_POST['type']==4){
$id=$_POST['id'];
$sql = "DELETE FROM student WHERE id in ($id)";
if (mysqli_query($conn, $sql)) {
echo $id;
}
else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
mysqli_close($conn);
}
}
?>
style.css
body {
color: #566787;
background: #f5f5f5;
font-family: varela round, sans-serif;
font-size: 13px;
}
.table-wrapper {
background: #fff;
padding: 20px 25px;
margin: 30px 0;
border-radius: 3px;
box-shadow: 0 1px 1px rgba(0, 0, 0, 0.05);
}
.table-title {
padding-bottom: 15px;
background: #721300;
color: #fff;
padding: 16px 30px;
margin: -20px -25px 10px;
border-radius: 3px 3px 0 0;
}
.table-title h2 {
margin: 5px 0 0;
font-size: 24px;
}
.table-title .btn-group {
float: right;
}
.table-title .btn {
color: #fff;
float: right;
font-size: 13px;
min-width: 50px;
border-radius: 2px;
border: none;
outline: none!important;
margin-left: 10px;
}
.table-title .btn i {
float: left;
font-size: 21px;
margin-right: 5px;
}
.table-title .btn span {
float: left;
margin-top: 2px;
}
table.table tr th,
table.table tr td {
border-color: #e9e9e9;
padding: 12px 15px;
vertical-align: middle;
}
table.table tr th:first-child {
width: 60px;
}
table.table tr th:last-child {
width: 100px;
}
table.table-striped tbody tr:nth-of-type(odd) {
background-color: #fcfcfc;
}
table.table-striped.table-hover tbody tr:hover {
background: #f5f5f5;
}
table.table th i {
font-size: 13px;
margin: 0 5px;
cursor: pointer;
}
table.table td:last-child i {
opacity: .9;
font-size: 22px;
margin: 0 5px;
}
table.table td a {
font-weight: 700;
color: #566787;
display: inline-block;
text-decoration: none;
outline: none !important;
}
table.table td a:hover {
color: #2196f3;
}
table.table td a.edit {
color: #ffc107;
}
table.table td a.delete {
color: #f44336;
}
table.table td i {
font-size: 19px;
}
table.table .avatar {
border-radius: 50%;
vertical-align: middle;
margin-right: 10px;
}
.pagination {
float: right;
margin: 0 0 5px;
}
.pagination li a {
border: none;
font-size: 13px;
min-width: 30px;
min-height: 30px;
color: #999;
margin: 0 2px;
line-height: 30px;
border-radius: 2px!important;
text-align: center;
padding: 0 6px;
}
.pagination li a:hover {
color: #666666;
}
.pagination li.active a,
.pagination li.active a.page-link {
background: #03a9f4;
}
.pagination li.active a:hover {
background: #0397d6;
}
.pagination li.disabled i {
color: #cccccc;
}
.pagination li i {
font-size: 16px;
padding-top: 6px;
}
.hint-text {
float: left;
margin-top: 10px;
font-size: 13px;
}
.custom-checkbox {
position: relative;
}
.custom-checkbox input[type=checkbox] {
opacity: 0;
position: absolute;
margin: 5px 0 0 3px;
z-index: 9;
}
.custom-checkbox label:before {
width: 18px;
height: 18px;
}
.custom-checkbox label:before {
content: '';
margin-right: 10px;
display: inline-block;
vertical-align: text-top;
background: #fff;
border: 1px solid #bbb;
border-radius: 2px;
box-sizing: border-box;
z-index: 2;
}
.custom-checkbox input[type=checkbox]:checked + label:after {
content: '';
position: absolute;
left: 6px;
top: 3px;
width: 6px;
height: 11px;
border: solid #000;
border-width: 0 3px 3px 0;
transform: inherit;
z-index: 3;
transform: rotateZ(45deg);
}
.custom-checkbox input[type=checkbox]:checked + label:before {
border-color: #03a9f4;
background: #03a9f4;
}
.custom-checkbox input[type=checkbox]:checked + label:after {
border-color: #ffffff;
}
.custom-checkbox input[type=checkbox]:disabled + label:before {
color: #b8b8b8;
cursor: auto;
box-shadow: none;
background: #dddddd;
}
.modal .modal-dialog {
max-width: 400px;
}
.modal .modal-header,
.modal .modal-body,
.modal .modal-footer {
padding: 20px 30px;
}
.modal .modal-content {
border-radius: 3px;
box-shadow: 0 1px 1px rgba(0, 0, 0, 0.05);
border:2px solid #dddddd;
}
.modal .modal-footer {
background: #ecf0f1;
border-radius: 0 0 3px 3px;
}
.modal .modal-title {
display: inline-block;
}
.modal .form-control {
border-radius: 2px;
box-shadow: none;
border-color: #dddddd;
}
.modal textarea.form-control {
resize: vertical;
}
.modal .btn {
border-radius: 2px;
min-width: 100px;
}
.modal form label {
font-weight: 400;
}
Demo
Download Source Code
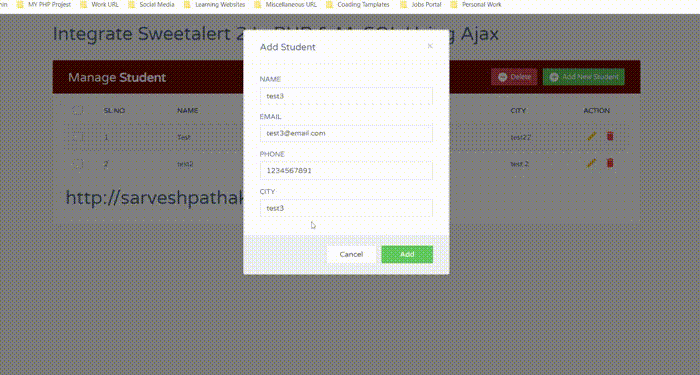