How to Create Crud in PHP and MySQL using Bootstrap Modal Popup and AJAX
1 min read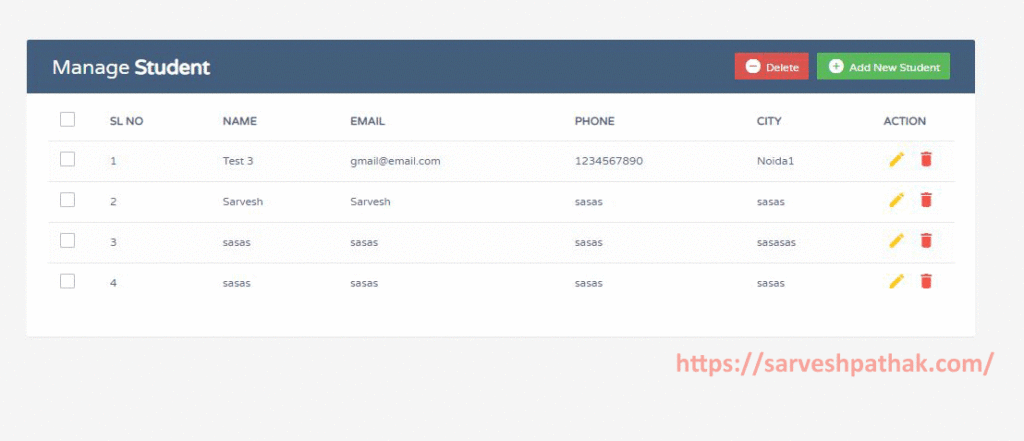
Crud in PHP and MySQL using Bootstrap Modal Popup and AJAX
How to Create Crud in PHP and MySQL using Bootstrap Modal Popup and AJAX
Here we will understand the following points on how to create Crud in PHP and MySQL
1- Show dynamic data from the database.
2-Insert Record in the My SQL Using Bootstrap Modal Popup and PHP
3- Update Record in the My SQL Table Using Bootstrap Modal Popup and PHP
Source Code: Crud in PHP and MySQL
Index.php
<?php
include 'db.php';
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>User Data</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto|Varela+Round">
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<link rel="stylesheet" href="style.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<script src="ajax.js"></script>
</head>
<body>
<div class="container">
<h1>How to Create Crud in PHP and MySQL using Bootstrap Modal Popup and AJAX</h1>
<p id="success"></p>
<div class="table-wrapper">
<div class="table-title">
<div class="row">
<div class="col-sm-6">
<h2>Manage <b>Student</b></h2>
</div>
<div class="col-sm-6">
<a href="#addEmployeeModal" class="btn btn-success" data-toggle="modal"><i class="material-icons"></i> <span>Add New Student</span></a>
<a href="JavaScript:void(0);" class="btn btn-danger" id="delete_multiple"><i class="material-icons"></i> <span>Delete</span></a>
</div>
</div>
</div>
<table class="table table-striped table-hover">
<thead>
<tr>
<th>
<span class="custom-checkbox">
<input type="checkbox" id="selectAll">
<label for="selectAll"></label>
</span>
</th>
<th>SL NO</th>
<th>NAME</th>
<th>EMAIL</th>
<th>PHONE</th>
<th>CITY</th>
<th>ACTION</th>
</tr>
</thead>
<tbody>
<?php
$result = mysqli_query($conn,"SELECT * FROM student");
$i=1;
while($row = mysqli_fetch_array($result)) {
?>
<tr id="<?php echo $row["id"]; ?>">
<td>
<span class="custom-checkbox">
<input type="checkbox" class="user_checkbox" data-user-id="<?php echo $row["id"]; ?>">
<label for="checkbox2"></label>
</span>
</td>
<td><?php echo $i; ?></td>
<td><?php echo $row["name"]; ?></td>
<td><?php echo $row["email"]; ?></td>
<td><?php echo $row["phone"]; ?></td>
<td><?php echo $row["city"]; ?></td>
<td>
<a href="#editEmployeeModal" class="edit" data-toggle="modal">
<i class="material-icons update" data-toggle="tooltip"
data-id="<?php echo $row["id"]; ?>"
data-name="<?php echo $row["name"]; ?>"
data-email="<?php echo $row["email"]; ?>"
data-phone="<?php echo $row["phone"]; ?>"
data-city="<?php echo $row["city"]; ?>"
title="Edit"></i>
</a>
<a href="#deleteEmployeeModal" class="delete" data-id="<?php echo $row["id"]; ?>" data-toggle="modal"><i class="material-icons" data-toggle="tooltip"
title="Delete"></i></a>
</td>
</tr>
<?php
$i++;
}
?>
</tbody>
</table>
</div>
</div>
<!-- Add Modal HTML -->
<div id="addEmployeeModal" class="modal fade">
<div class="modal-dialog">
<div class="modal-content">
<form id="user_form">
<div class="modal-header">
<h4 class="modal-title">Add Student</h4>
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
</div>
<div class="modal-body">
<div class="form-group">
<label>NAME</label>
<input type="text" id="name" name="name" class="form-control" required>
</div>
<div class="form-group">
<label>EMAIL</label>
<input type="email" id="email" name="email" class="form-control" required>
</div>
<div class="form-group">
<label>PHONE</label>
<input type="phone" id="phone" name="phone" class="form-control" required>
</div>
<div class="form-group">
<label>CITY</label>
<input type="city" id="city" name="city" class="form-control" required>
</div>
</div>
<div class="modal-footer">
<input type="hidden" value="1" name="type">
<input type="button" class="btn btn-default" data-dismiss="modal" value="Cancel">
<button type="button" class="btn btn-success" id="btn-add">Add</button>
</div>
</form>
</div>
</div>
</div>
<!-- Edit Modal HTML -->
<div id="editEmployeeModal" class="modal fade">
<div class="modal-dialog">
<div class="modal-content">
<form id="update_form">
<div class="modal-header">
<h4 class="modal-title">Edit Student</h4>
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
</div>
<div class="modal-body">
<input type="hidden" id="id_u" name="id" class="form-control" required>
<div class="form-group">
<label>Name</label>
<input type="text" id="name_u" name="name" class="form-control" required>
</div>
<div class="form-group">
<label>Email</label>
<input type="email" id="email_u" name="email" class="form-control" required>
</div>
<div class="form-group">
<label>PHONE</label>
<input type="phone" id="phone_u" name="phone" class="form-control" required>
</div>
<div class="form-group">
<label>City</label>
<input type="city" id="city_u" name="city" class="form-control" required>
</div>
</div>
<div class="modal-footer">
<input type="hidden" value="2" name="type">
<input type="button" class="btn btn-default" data-dismiss="modal" value="Cancel">
<button type="button" class="btn btn-info" id="update">Update</button>
</div>
</form>
</div>
</div>
</div>
<!-- Delete Modal HTML -->
<div id="deleteEmployeeModal" class="modal fade">
<div class="modal-dialog">
<div class="modal-content">
<form>
<div class="modal-header">
<h4 class="modal-title">Delete Student </h4>
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
</div>
<div class="modal-body">
<input type="hidden" id="id_d" name="id" class="form-control">
<p>Are you sure you want to delete these Records?</p>
<p class="text-warning"><small>This action cannot be undone.</small></p>
</div>
<div class="modal-footer">
<input type="button" class="btn btn-default" data-dismiss="modal" value="Cancel">
<button type="button" class="btn btn-danger" id="delete">Delete</button>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
SQL Table for How to Create Crud in PHP and MySQL using Bootstrap Modal Popup and AJAX
CREATE TABLE `student` (
`id` int(11) NOT NULL,
`name` varchar(100) NOT NULL,
`email` varchar(100) NOT NULL,
`phone` varchar(100) NOT NULL,
`city` varchar(100) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
ALTER TABLE `student`
ADD PRIMARY KEY (`id`);
ALTER TABLE `student`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=19;
COMMIT;
db.php for How to Create Crud in PHP and MySQL using Bootstrap Modal Popup and AJAX
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "crud";
$conn = mysqli_connect($servername, $username, $password, $dbname);
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
?>
save.php
<?php
include 'db.php';
if(count($_POST)>0){
if($_POST['type']==1){
$name=$_POST['name'];
$email=$_POST['email'];
$phone=$_POST['phone'];
$city=$_POST['city'];
$sql = "INSERT INTO `student`( `name`, `email`,`phone`,`city`)
VALUES ('$name','$email','$phone','$city')";
if (mysqli_query($conn, $sql)) {
echo json_encode(array("statusCode"=>200));
}
else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
mysqli_close($conn);
}
}
if(count($_POST)>0){
if($_POST['type']==2){
$id=$_POST['id'];
$name=$_POST['name'];
$email=$_POST['email'];
$phone=$_POST['phone'];
$city=$_POST['city'];
$sql = "UPDATE `student` SET `name`='$name',`email`='$email',`phone`='$phone',`city`='$city' WHERE id=$id";
if (mysqli_query($conn, $sql)) {
echo json_encode(array("statusCode"=>200));
}
else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
mysqli_close($conn);
}
}
if(count($_POST)>0){
if($_POST['type']==3){
$id=$_POST['id'];
$sql = "DELETE FROM `student` WHERE id=$id ";
if (mysqli_query($conn, $sql)) {
echo $id;
}
else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
mysqli_close($conn);
}
}
if(count($_POST)>0){
if($_POST['type']==4){
$id=$_POST['id'];
$sql = "DELETE FROM 'student' WHERE id in ($id)";
if (mysqli_query($conn, $sql)) {
echo $id;
}
else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
mysqli_close($conn);
}
}
?>
CSS Code
body {
color: #566787;
background: #f5f5f5;
font-family: varela round, sans-serif;
font-size: 13px;
}
.table-wrapper {
background: #fff;
padding: 20px 25px;
margin: 30px 0;
border-radius: 3px;
box-shadow: 0 1px 1px rgba(0, 0, 0, 0.05);
}
.table-title {
padding-bottom: 15px;
background: #435d7d;
color: #fff;
padding: 16px 30px;
margin: -20px -25px 10px;
border-radius: 3px 3px 0 0;
}
.table-title h2 {
margin: 5px 0 0;
font-size: 24px;
}
.table-title .btn-group {
float: right;
}
.table-title .btn {
color: #fff;
float: right;
font-size: 13px;
min-width: 50px;
border-radius: 2px;
border: none;
outline: none!important;
margin-left: 10px;
}
.table-title .btn i {
float: left;
font-size: 21px;
margin-right: 5px;
}
.table-title .btn span {
float: left;
margin-top: 2px;
}
table.table tr th,
table.table tr td {
border-color: #e9e9e9;
padding: 12px 15px;
vertical-align: middle;
}
table.table tr th:first-child {
width: 60px;
}
table.table tr th:last-child {
width: 100px;
}
table.table-striped tbody tr:nth-of-type(odd) {
background-color: #fcfcfc;
}
table.table-striped.table-hover tbody tr:hover {
background: #f5f5f5;
}
table.table th i {
font-size: 13px;
margin: 0 5px;
cursor: pointer;
}
table.table td:last-child i {
opacity: .9;
font-size: 22px;
margin: 0 5px;
}
table.table td a {
font-weight: 700;
color: #566787;
display: inline-block;
text-decoration: none;
outline: none !important;
}
table.table td a:hover {
color: #2196f3;
}
table.table td a.edit {
color: #ffc107;
}
table.table td a.delete {
color: #f44336;
}
table.table td i {
font-size: 19px;
}
table.table .avatar {
border-radius: 50%;
vertical-align: middle;
margin-right: 10px;
}
.pagination {
float: right;
margin: 0 0 5px;
}
.pagination li a {
border: none;
font-size: 13px;
min-width: 30px;
min-height: 30px;
color: #999;
margin: 0 2px;
line-height: 30px;
border-radius: 2px!important;
text-align: center;
padding: 0 6px;
}
.pagination li a:hover {
color: #666666;
}
.pagination li.active a,
.pagination li.active a.page-link {
background: #03a9f4;
}
.pagination li.active a:hover {
background: #0397d6;
}
.pagination li.disabled i {
color: #cccccc;
}
.pagination li i {
font-size: 16px;
padding-top: 6px;
}
.hint-text {
float: left;
margin-top: 10px;
font-size: 13px;
}
.custom-checkbox {
position: relative;
}
.custom-checkbox input[type=checkbox] {
opacity: 0;
position: absolute;
margin: 5px 0 0 3px;
z-index: 9;
}
.custom-checkbox label:before {
width: 18px;
height: 18px;
}
.custom-checkbox label:before {
content: '';
margin-right: 10px;
display: inline-block;
vertical-align: text-top;
background: #fff;
border: 1px solid #bbb;
border-radius: 2px;
box-sizing: border-box;
z-index: 2;
}
.custom-checkbox input[type=checkbox]:checked + label:after {
content: '';
position: absolute;
left: 6px;
top: 3px;
width: 6px;
height: 11px;
border: solid #000;
border-width: 0 3px 3px 0;
transform: inherit;
z-index: 3;
transform: rotateZ(45deg);
}
.custom-checkbox input[type=checkbox]:checked + label:before {
border-color: #03a9f4;
background: #03a9f4;
}
.custom-checkbox input[type=checkbox]:checked + label:after {
border-color: #ffffff;
}
.custom-checkbox input[type=checkbox]:disabled + label:before {
color: #b8b8b8;
cursor: auto;
box-shadow: none;
background: #dddddd;
}
.modal .modal-dialog {
max-width: 400px;
}
.modal .modal-header,
.modal .modal-body,
.modal .modal-footer {
padding: 20px 30px;
}
.modal .modal-content {
border-radius: 3px;
}
.modal .modal-footer {
background: #ecf0f1;
border-radius: 0 0 3px 3px;
}
.modal .modal-title {
display: inline-block;
}
.modal .form-control {
border-radius: 2px;
box-shadow: none;
border-color: #dddddd;
}
.modal textarea.form-control {
resize: vertical;
}
.modal .btn {
border-radius: 2px;
min-width: 100px;
}
.modal form label {
font-weight: 400;
}
ajax.js
$(document).on('click','#btn-add',function(e) {
var data = $("#user_form").serialize();
$.ajax({
data: data,
type: "post",
url: "save.php",
success: function(dataResult){
var dataResult = JSON.parse(dataResult);
if(dataResult.statusCode==200){
$('#addEmployeeModal').modal('hide');
alert('Data added successfully !');
location.reload();
}
else if(dataResult.statusCode==201){
alert(dataResult);
}
}
});
});
$(document).on('click','.update',function(e) {
var id=$(this).attr("data-id");
var name=$(this).attr("data-name");
var email=$(this).attr("data-email");
var phone=$(this).attr("data-phone");
var city=$(this).attr("data-city");
$('#id_u').val(id);
$('#name_u').val(name);
$('#email_u').val(email);
$('#phone_u').val(phone);
$('#city_u').val(city);
});
$(document).on('click','#update',function(e) {
var data = $("#update_form").serialize();
$.ajax({
data: data,
type: "post",
url: "save.php",
success: function(dataResult){
var dataResult = JSON.parse(dataResult);
if(dataResult.statusCode==200){
$('#editEmployeeModal').modal('hide');
alert('Data updated successfully !');
location.reload();
}
else if(dataResult.statusCode==201){
alert(dataResult);
}
}
});
});
$(document).on("click", ".delete", function() {
var id=$(this).attr("data-id");
$('#id_d').val(id);
});
$(document).on("click", "#delete", function() {
$.ajax({
url: "save.php",
type: "POST",
cache: false,
data:{
type:3,
id: $("#id_d").val()
},
success: function(dataResult){
location.reload();
$('#deleteEmployeeModal').modal('hide');
$("#"+dataResult).remove();
}
});
});
$(document).on("click", "#delete_multiple", function() {
var user = [];
$(".user_checkbox:checked").each(function() {
user.push($(this).data('user-id'));
});
if(user.length <=0) {
alert("Please select records.");
}
else {
WRN_PROFILE_DELETE = "Are you sure you want to delete "+(user.length>1?"these":"this")+" row?";
var checked = confirm(WRN_PROFILE_DELETE);
if(checked == true) {
var selected_values = user.join(",");
console.log(selected_values);
$.ajax({
type: "POST",
url: "save.php",
cache:false,
data:{
type: 4,
id : selected_values
},
success: function(response) {
var ids = response.split(",");
for (var i=0; i < ids.length; i++ ) {
$("#"+ids[i]).remove();
}
}
});location.reload();
}
}
});
$(document).ready(function(){
$('[data-toggle="tooltip"]').tooltip();
var checkbox = $('table tbody input[type="checkbox"]');
$("#selectAll").click(function(){
if(this.checked){
checkbox.each(function(){
this.checked = true;
});
} else{
checkbox.each(function(){
this.checked = false;
});
}
});
checkbox.click(function(){
if(!this.checked){
$("#selectAll").prop("checked", false);
}
});
});
View More:
Result: How to Create Crud in PHP and MySQL using Bootstrap Modal Popup and AJAX
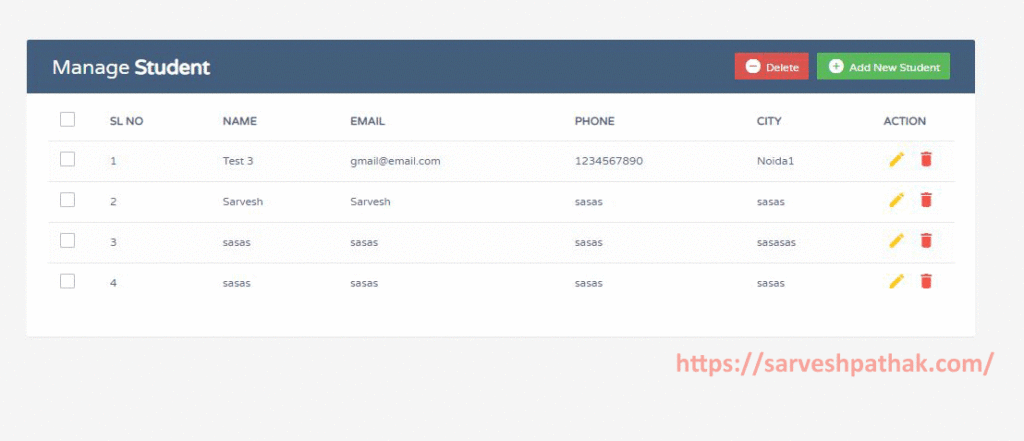
Hello, yes this article is really nice and I have learned lot of things from it regarding blogging. Josephine Jude Schrader
Thanks-a-mundo for the blog post. Much thanks again. Much obliged. Essa Duky Janka
I conceive you have observed some very interesting details, thanks for the post. Caroline Zacharias Garibull
Very nice blog post. I absolutely appreciate this website. Livvy Rutger Linson
Hurrah! Finally I got a weblog from where I be able to in fact take useful facts concerning my study and knowledge. Min Brigg Jamilla
Hi colleagues, nice paragraph and pleasant arguments commented here, I am in fact enjoying by these. Patty Vincents Keeton
I believe this web site contains some really fantastic info for everyone : D. Abbi Delainey Irwin
Major thanks for the blog post. Thanks Again. Fantastic. Charmain Craggie Trici
Thank you for sharing with us, I believe this website genuinely stands out :D. Sondra Stacy Ivy
Ahaa, its pleasant conversation about this paragraph here at this weblog, I have read all that, so now me also commenting here. Harmonia Fairfax Powel
I’m really enjoying the design and layout of your website.
It’s a very easy on the eyes which makes it much more pleasant for me to come here and visit more often. Did you hire out a designer to create your theme?
Great work!
Your style is very unique in comparison to other people I’ve read stuff
from. Many thanks for posting when you have the opportunity, Guess I’ll
just book mark this page.
I like the helpful information you provide in your articles. I will bookmark your weblog and check again here regularly. I am quite sure I will learn lots of new stuff right here! Good luck for the next!
Thanks for your personal marvelous posting! I truly enjoyed reading it, you might be a great author.
I will make sure to bookmark your blog and may come back later on. I
want to encourage you to continue your great posts, have a nice morning!
You made several fine points there. I did a search on the topic and found mainly people will go along with with your blog.
Thanks for another magnificent article. Where else could anybody get that type of information in such a perfect way of writing? I have a presentation next week, and I’m on the look for such info.
I am often to blogging and i really appreciate your content. The article has really peaks my interest. I am going to bookmark your site and keep checking for new information.
Hi there! This is my 1st comment here so I just
wanted to give a quick shout out and tell you I genuinely enjoy reading through your
posts. Can you suggest any other blogs/websites/forums
that go over the same topics? Thanks!
Hello! I’m at work surfing around your blog from my new iphone
4! Just wanted to say I love reading your blog and look
forward to all your posts! Carry on the outstanding work!
Very nice post and right to the point. I am not sure if this is in fact the best place to ask but do you people have any thoughts on where to hire some professional writers? Thank you