How to Create and Consume RESTful JSON Web Service in PHP
1 min read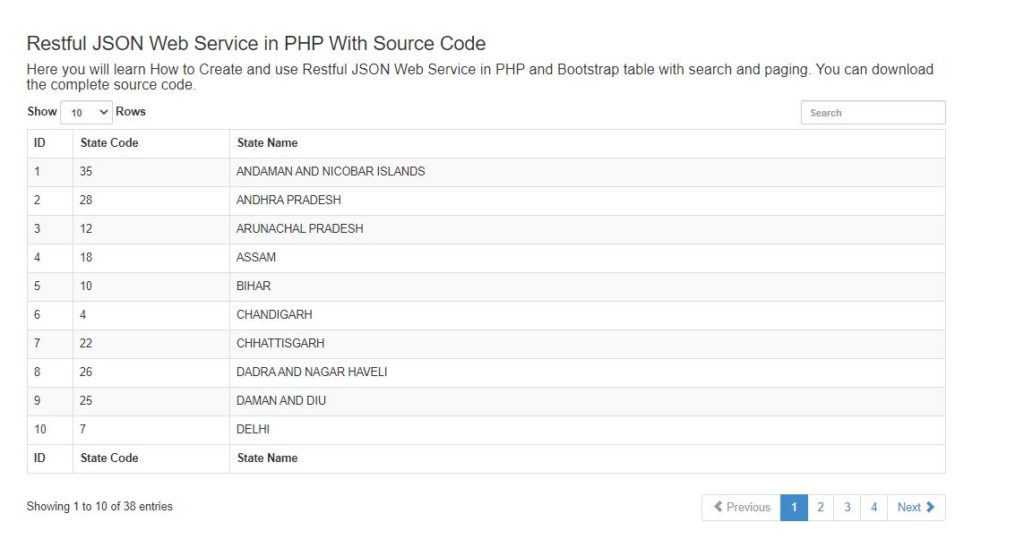
How to Create and Consume RESTful JSON Web Service in PHP
What are RESTful web services?
RESTful web services are built to work best on the Web. Representational State Transfer (REST) is an architectural style that specifies constraints, such as the uniform interface, that if applied to a web service induce desirable properties, such as performance, scalability, and modifiability, that enable services to work best on the Web.
In the REST architectural style, data and functionality are considered resources and are accessed using Uniform Resource Identifiers (URIs), typically links on the Web. The resources are acted upon by using a set of simple, well-defined operations. The REST architectural style constrains an architecture to a client/server architecture and is designed to use a stateless communication protocol, typically HTTP.
In the REST architecture style, clients and servers exchange representations of resources by using a standardized interface and protocol.
Here is an example of How to Create and Consume RESTful JSON Web Service in PHP and Bootstrap table with search and paging. You can download the complete source code.
webservice.php
<?php
define('DB_SERVER','localhost');
define('DB_USER','root');
define('DB_PASS' ,'');
define('DB_NAME', 'india');
$con = mysqli_connect(DB_SERVER,DB_USER,DB_PASS,DB_NAME);
if (mysqli_connect_errno())
{
echo "Failed to connect to MySQL: " . mysqli_connect_error();
}
$qur = "SELECT id, state_code , state_name FROM india_state";
$qur=mysqli_query($con,$qur);
$result =array();
while($r = mysqli_fetch_array($qur)){
extract($r);
$result[] = array('id' => $id, 'state_code' => $state_code, 'state_name' => $state_name);
}
$json = $result;
@mysqli_close($con);
header('Content-type: application/json');
echo json_encode($json);
?>
Now the RESTful JSON Web Service is Created. Then create a page to consume the web service
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="UTF-8">
<title>How to Create and Consume RESTful JSON Web Service in PHP</title>
<link rel='stylesheet' href='https://netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap.min.css'><link rel="stylesheet" href="./style.css">
</head>
<body>
<!-- partial:index.partial.html -->
<div class="container demo">
<table class="datatable table table-striped table-bordered">
<thead>
<tr>
<th>ID</th>
<th>State Code</th>
<th>State Name</th>
</tr>
</thead>
<tbody>
<?php
$url ="http://localhost:8013/rest/webservice.php";
$client = curl_init($url);
curl_setopt($client,CURLOPT_RETURNTRANSFER,true);
$response = curl_exec($client);
$json_data = file_get_contents($url);
$response_data = json_decode($json_data);
foreach ($response_data as $user) {
echo "<tr><td>".$user->id."</td> <td>".$user->state_code ."</td><td>".$user->state_name ."</td></tr>";
}
?>
</tbody>
<tfoot>
<tr>
<th>ID</th>
<th>State Code</th>
<th>State Name</th>
</tr>
</tfoot>
</table>
</div><!--/.container.demo -->
<!-- partial -->
<script src='https://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js'></script>
<script src='https://netdna.bootstrapcdn.com/bootstrap/3.1.1/js/bootstrap.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/datatables/1.9.4/jquery.dataTables.min.js'></script><script src="./script.js"></script>
</body>
</html>
The output of Create and Consume RESTful JSON Web Service in PHP
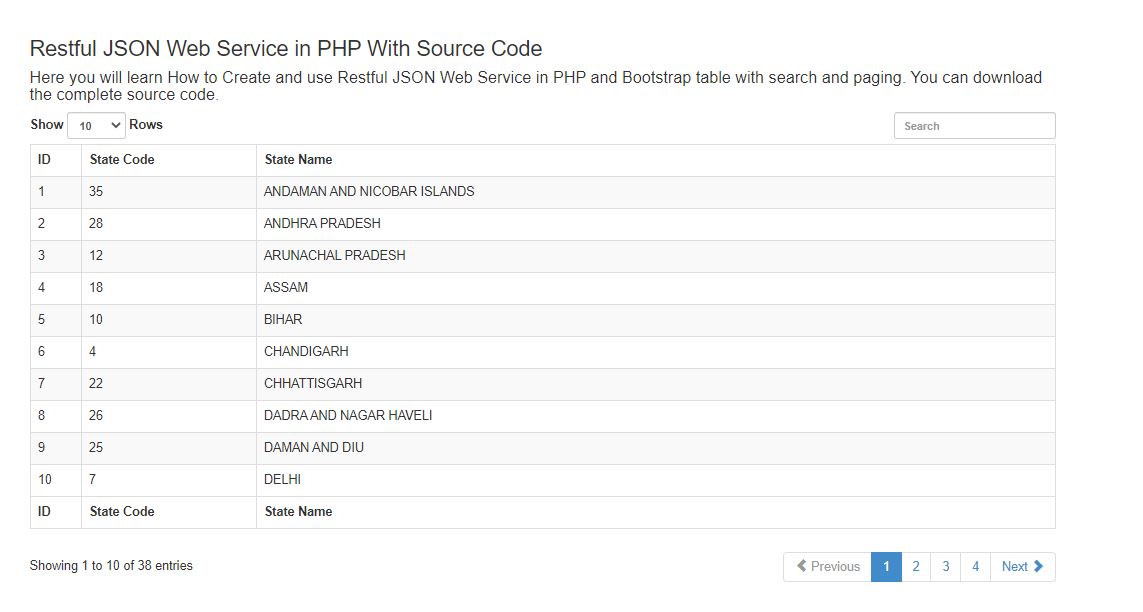
I was extremely pleased to find this web site.
I wanted to thank you for your time for this particularly wonderful read!!
I definitely enjoyed every part of it and
I have you bookmarked to see new things on your website.
Review my web site; Royal CBD