How to Dynamically Add / Remove row with multiple input fields in PHP Form
2 min read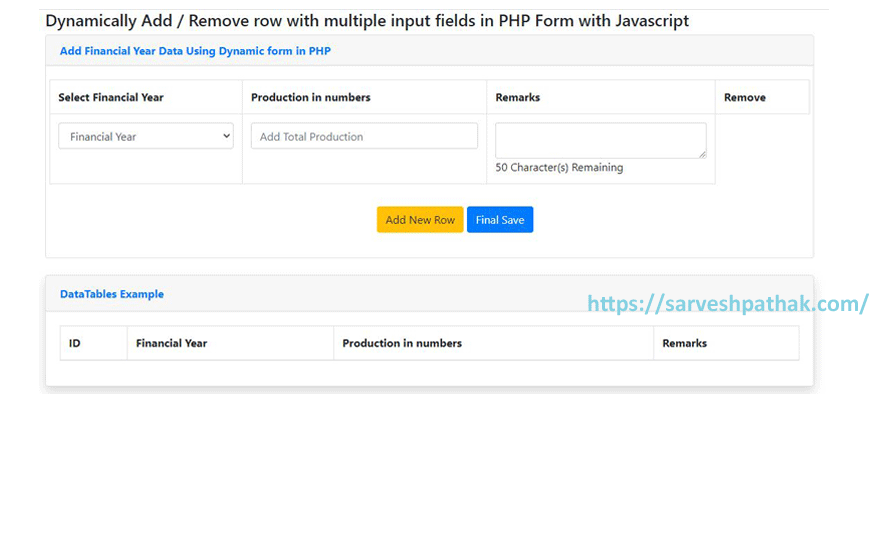
Dynamically Add / Remove row with multiple input
How to Dynamically Add / Remove row with multiple input fields in PHP Form
Here we will understand the following points on how to Dynamically Add / Remove rows with multiple input fields in PHP Form using Java Script
1- How to Create Add New Row Feature in the Form
2-How to Create Dynamic form with a Dynamic Dropdown
3- How to Insert Data in MySQL Table from Dynamic Form
4- How to Create Dynamic Character Counter in the Text area
4- How to Show data in Tables
MySQL Table structure Dynamically Add / Remove row with multiple input fields
--
-- Table structure for table `mis_production`
--
CREATE TABLE `mis_production` (
`sr_id` int(11) NOT NULL,
`fin_year` varchar(20) NOT NULL,
`production` int(11) NOT NULL,
`remarks` varchar(60) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Table structure for table `mis_year`
--
CREATE TABLE `mis_year` (
`sr_id` int(11) NOT NULL,
`fin_year` varchar(20) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
Dynamically Add / Remove row with multiple input fields in PHP Form using Javascript
Connect Database
define('DB_SERVER','localhost');
define('DB_USER','root');
define('DB_PASS' ,'');
define('DB_NAME', 'school');
$con = mysqli_connect(DB_SERVER,DB_USER,DB_PASS,DB_NAME);
Create PHP Method to Bind Dropdown
function fill_year_select_box($con)
{
$output = '';
$query=mysqli_query($con,"SELECT fin_year FROM mis_year");
$cnt=1;
while($row=mysqli_fetch_array($query))
{
$output .= " $row[0] ";
}
return $output;
}
Dynamically Add / Remove row with multiple input fields in PHP Form using Javascript
Create JavaScript Method To calculate Text Length
<script>
//Method to show remaning text
function countChar(val,$msg,$ct) {
$text1=count_message;
var len = val.value.length;
if (len >= $ct) {
val.value = val.value.substring(0, $ct);
} else {
$($msg).text($ct - len);
}
};
</script>
JavaScript Method to Create a New Row
<script type="text/javascript">
// Method to create new Row
function add_row()
{
$rowno=$("#employee_table tr").length;
$rowno=$rowno+1;
$second="<td>"+$rowno-1+"</td><td><select name='financial_year[]' class='form-control' required><option value=''>Financial Year</option> <?php echo fill_year_select_box($con);?></select></td>";
$fourth="<td><input type='number' class='form-control' name='production[]' placeholder=' Add Total Production'/></td>";
$fifth="<td><textarea rows = '2' cols = '20' onkeyup='countChar(this,count_message"+$rowno+",50)' id='remarks' name='remarks[]' class='char-counter maxlength='50' form-control form-control-sm' rows='2' placeholder='Remarks' > </textarea><br/><span class='pull-right label label-default' id='count_message"+$rowno+"'>50</span> Character(s) Remaining </td>";
$("#employee_table tr:last").after("<tr id='row"+$rowno+"' class='border-bottom-primary'>"+$second+""+$fourth+""+$fifth+" <td><a href='#' class='btn btn-danger btn-circle btn-sm' onclick=delete_row('row"+$rowno+"')><i class='fas fa-trash'></i>Remove</a> </td></tr>");
}
function delete_row(rowno)
{
$('#'+rowno).remove();
}
</script>
Dynamically Add / Remove row with multiple input fields in PHP Form using Javascript
Create Form in PHP
<form class="form-horizontal" name="insertstore" method="post" enctype="multipart/form-data">
<?php echo $msg; ?>
<div class="form-group row">
<table id="employee_table" class="table table-bordered dataTable" align=center>
<tr ><th class="border-bottom-primary"> Select Financial Year</th><th class="border-bottom-primary">Production in numbers</th><th class="border-bottom-primary"> Remarks </th> <th rowspan="1" > Remove</th> </tr>
<tr id="row1" class="border-bottom-primary">
<td>
<select id="financial_year" name="financial_year[]" class="form-control" required>
<option value="">Financial Year</option>
<?php echo fill_year_select_box($con);?>
</select>
</td>
<td><input type="number" name="production[]"class="form-control" placeholder="Add Total Production"> </td>
<td>
<textarea rows = "2" cols = "20" onkeyup="countChar(this,count_message,50)" maxlength="50" id="remarks" name="remarks[]" class="char-counter form-control form-control-sm" rows="2" placeholder="Remarks" > </textarea>
<span class="pull-right label label-default" id="count_message">50</span> Character(s) Remaining
</td>
</tr>
</table>
</div>
<div class="form-group row">
<label class="col-sm-5 col-form-label" for="inputLastName"></label>
<div class="col-sm-6">
<input class="btn btn-warning" type="button" onclick="add_row();" value="Add New Row">
<button type="submit" name="submit" class="btn btn-primary">Final Save</button></div>
</div>
</form>
Save Form Data
<?php
if(isset($_POST['submit']))
{
$itemCount = count($_POST["financial_year"]);
$query = "INSERT INTO mis_production (fin_year, production,remarks) VALUES ";
$queryValue = "";
for($i=0;$i<$itemCount;$i++) {
if(!empty($_POST["production"][$i]) || !empty($_POST["financial_year"][$i])) {
$itemValues++;
if($queryValue!="") {
$queryValue .= ",";
}
$queryValue .= "('" . $_POST["financial_year"][$i] . "', " . $_POST["production"][$i] . ", '" . $_POST["remarks"][$i] . "')";
}
}
$sql = $query.$queryValue;
if($itemValues!=0) {
$result = mysqli_query($con, $sql);
if(!empty($result)) $message = "Added Successfully.";
$msg="<div class='alert alert-success '><strong>Welcome!".$row['sr_id']."</strong> Added Successfully<button type='button' class='close' data-dismiss='alert'>×</button> </div>";
}
}
?>
Show Saved Records
<table class="table table-bordered" id="dataTable" width="100%" cellspacing="0">
<thead>
<tr>
<th>ID</th>
<th>Financial Year</th>
<th>Production in numbers</th>
<th>Remarks</th>
</tr>
</thead>
<tbody>
<?php
$command="SELECT * from mis_production ";
$query=mysqli_query($con,$command);
$cnt=1;
while($row=mysqli_fetch_array($query))
{
?>
<tr>
<td><?php echo htmlentities($row['sr_id']);?></td>
<td><?php echo htmlentities($row['fin_year']);?></td>
<td><?php echo htmlentities($row['production']);?></td>
<td><?php echo htmlentities($row['remarks']);?></td>
</tr>
<?php }?>
</tbody>
</table>
Dynamically Add / Remove row with multiple input fields in PHP Form using Javascript
Download Source Code Click Me
View Demo
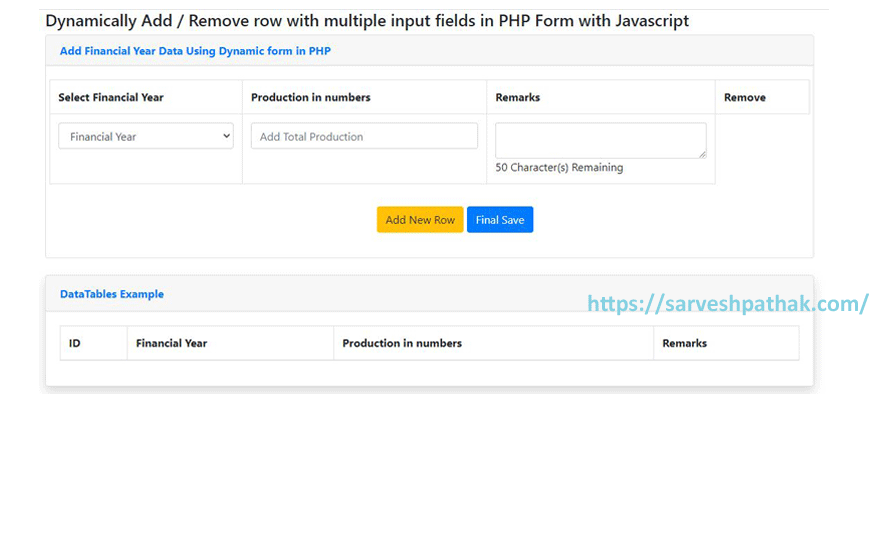
you have an excellent blog site below! would certainly you such as to make some invite blog posts on my blog site? Esther Emmy Clancy
As a Newbie, I am continuously exploring online for articles that can be of assistance to me. Thank you
As a Newbie, I am permanently exploring online for articles that can benefit me. Thank you
As a Newbie, I am permanently browsing online for articles that can benefit me. Thank you
Superb site you have here but I was wondering if you knew of any user discussion forums that cover the same topics discussed here?
I’d really love to be a part of group where I can get advice from other knowledgeable individuals that share the same interest.
If you have any recommendations, please let me know.
Many thanks!
Thanks-a-mundo for the article post. Keep writing. Tara Allin Areta
Excellent article. I definitely love this site. Thanks! Sella Marlo Lange
Generally I do not read article on blogs, but I would like to say that this write-up very forced me to take a look at and do it! Your writing taste has been surprised me. Thanks, quite nice post. Clarinda Emile Yablon
Excellent article. I’m going through a few of these issues as well..